In the world of frontend development, handling external data especially from APIs can often lead to unexpected runtime errors. While TypeScript offers compile-time type safety, it falls short when dealing with unknown data. This is where Zod Documentation becomes invaluable. Zod is a powerful library that simplifies schema validations, ensuring that incoming data adheres to a predefined structure.
By using Zod documentation for schema validations, developers can prevent errors before they reach the application runtime, ensuring both data integrity and a smoother user experience. In this guide, we’ll explore how Zod Documentation for schema validation, intuitive API and robust features can enhance data validation, making your application more resilient and reliable.
Why is Schema Validation Important for Frontend Developers?
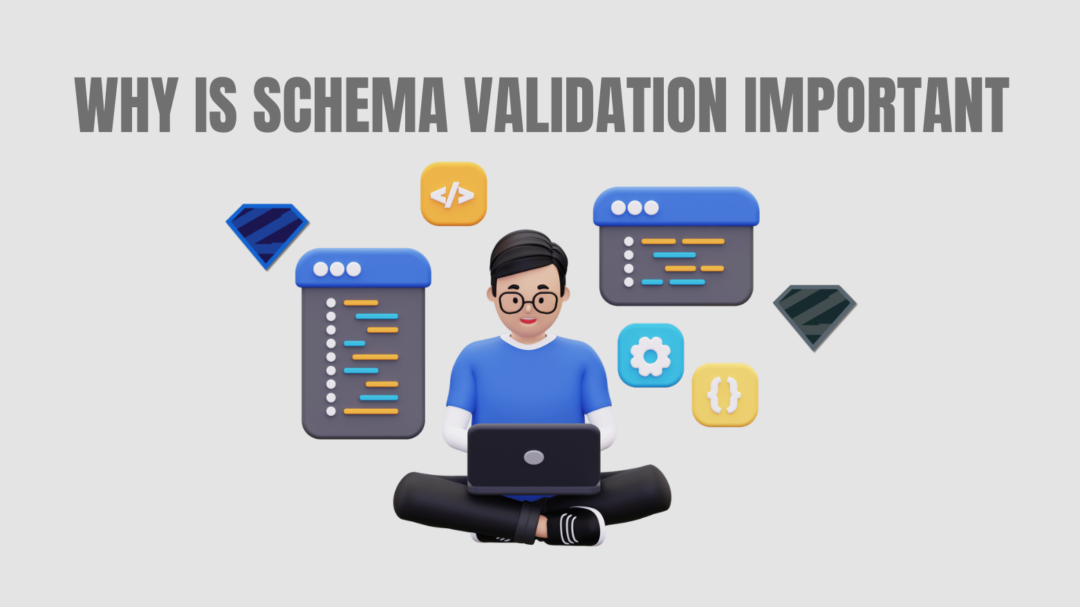
As a Frontend Developer, you’re no stranger to the chaos of handling data coming from APIs. It’s one of the core challenges of building modern applications working with data you don’t control. While tools like TypeScript help prevent some common runtime errors, they don’t provide full protection against the unpredictable nature of external data.
TypeScript offers compile-time safety by enforcing strict type definitions. But when it comes to working with unknown data such as API responses TypeScript alone doesn’t fully mitigate the risk of runtime errors. This is where Zod, a powerful schema validation library, comes into play. Zod can help reduce these runtime errors by ensuring that incoming data adheres to a predefined schema, safeguarding your application from unexpected bugs.
Basic Usage of Zod Schema Validation
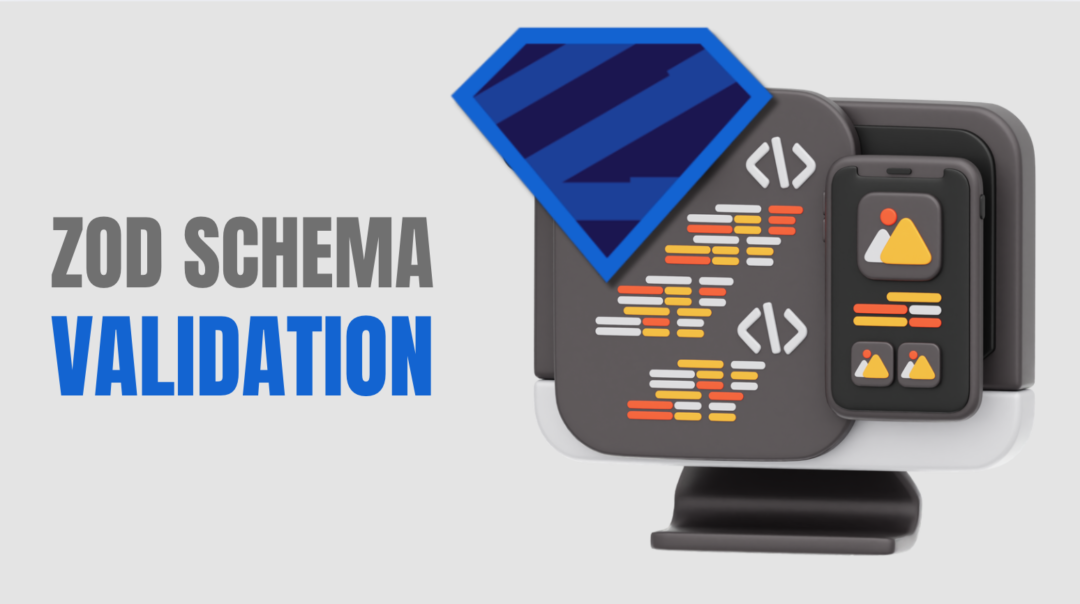
Installation
First, you need to install Zod if you haven’t already:
npm install zod
Creating a Schema
In Zod, you define a schema using a set of methods that represent different data types. Here’s an example of a basic validation schema for a user object.
import { z } from 'zod';
const UserSchema = z.object({
name: z.string().min(3), // Name must be a string with at least 3 characters
age: z.number().int().min(18), // Age must be an integer and at least 18
email: z.string().email(), // Email must be a valid email address
});
Validating Data
Once the schema is created, you can validate data by calling the parse
method:
try {
const userData = {
name: 'John',
age: 25,
email: 'john.doe@example.com'
};
// Validate the data
const validatedData = UserSchema.parse(userData);
console.log(validatedData); // This will print the validated data
} catch (error) {
if (error instanceof z.ZodError) {
console.log(error.errors); // Prints an array of validation errors
}
}
Type Inference
Zod leverages TypeScript’s type system, so you get full type inference based on the schema definition:
type User = z.infer<typeof UserSchema>;
const user: User = {
name: 'Alice',
age: 22,
email: 'alice@example.com'
};
The User
type here is automatically inferred from the UserSchema
.
Typescript: Productivity Over Safety
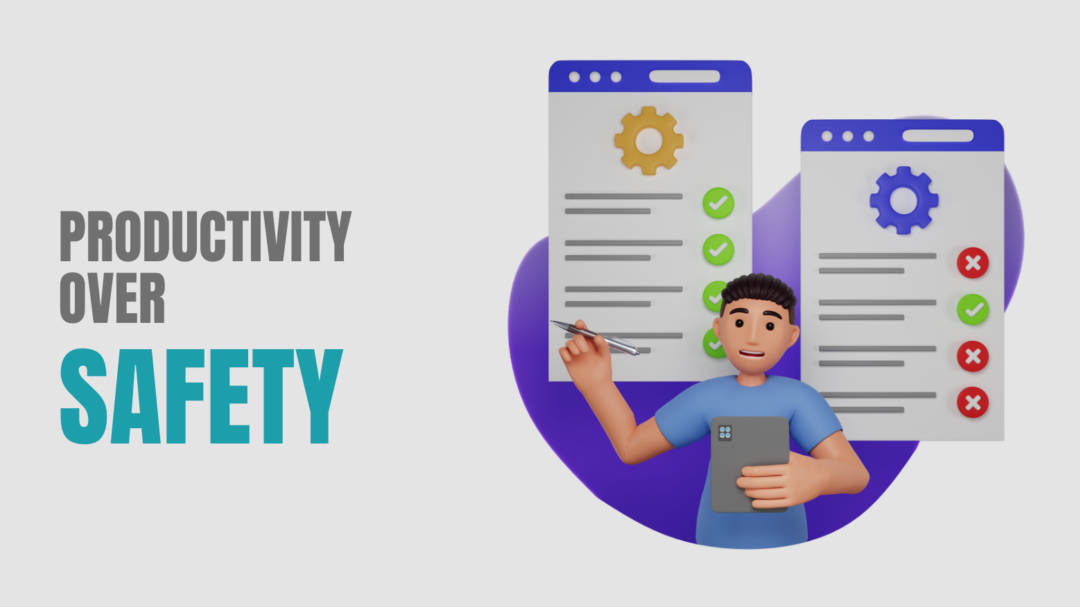
With TypeScript, developers often focus on productivity over safety. Sure, TypeScript highlights unexpected behavior, making it easier to catch bugs during development, but there are still gaps in handling external data. Consider the infamous use of any
and unknown
in TypeScript:
any
: Whileany
can be useful in certain scenarios, it essentially disables type-checking for that variable. For example, usingJSON.parse()
returnsany
, meaning TypeScript won’t validate the parsed object, opening up a world of potential runtime errors.
const myVar: MyType = JSON.parse(input); // No type safety
unknown
: Replacingany
withunknown
is an improvement, but it’s still a compromise. It forces developers to assert the type explicitly, which is better than no validation, but doesn’t completely address the problem of external data validation.
const myVar = JSON.parse(input) as MyType; // Type assertion
Enter Zod: The Ultimate Schema Validation Tool
When you hear the name “Zod,” it’s hard not to think of Superman’s nemesis. But, just like the villain Zod, Zod the library is a force to be reckoned with in the world of schema validation. With its agility and power, Zod makes it easier to ensure the runtime validity of external data, reducing the risk of unexpected bugs.
At its core, Zod is a lightweight and schema validation tool. It enables you to define schemas that describe the shape of your data and validates incoming data against these schemas. This adds an additional layer of safety on top of TypeScript’s static checks, making your code more robust and reliable.
Defining Your Schema with Zod
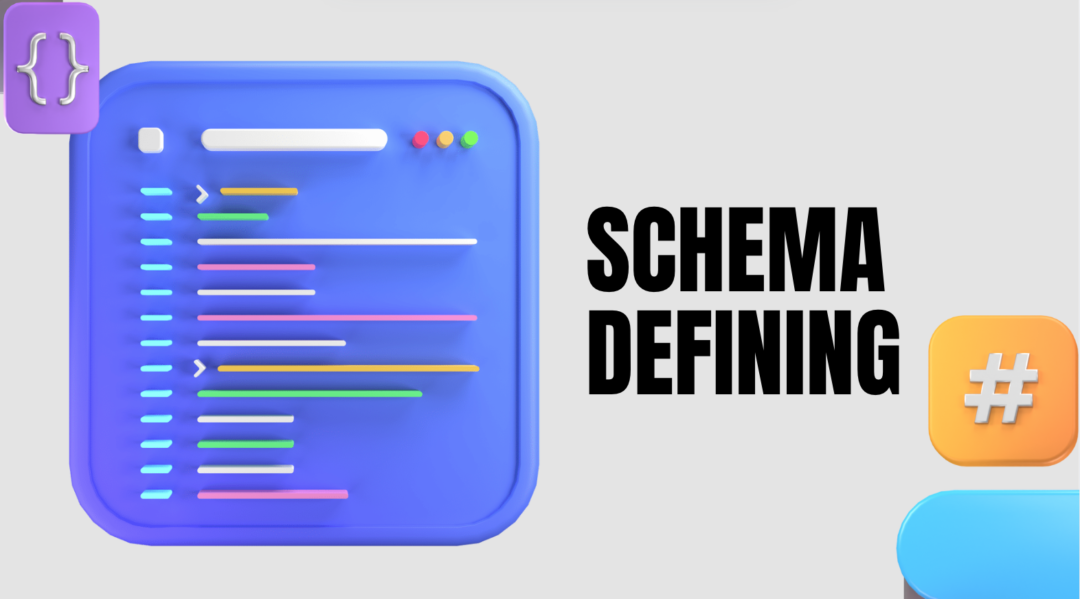
The first step in using Zod is defining your schema. Whether your data is simple or complex, Zod provides an easy-to-use API to define schemas that reflect your expected data structure. Here’s a basic example of how to define a schema in Zod:
import * as z from "zod";
const dataSchema = z.object({
data: z.record(
z.string(),
z.object({
id: z.string(),
meta: z.object({
name: z.string().optional(),
code: z.string(),
type: z.string(),
}),
})
),
});
In this example, we’re defining a schema for an object (
) with a key z.optional()
data
that holds a record of objects. Zod provides support for primitive types, optional values, unions, and even native enums, making it extremely flexible.
Zod also allows for more complex refinements, such as making fields optional (.optional()
) or validating the length of strings. You can define complex data structures with just a few lines of code.
Validating Your Data
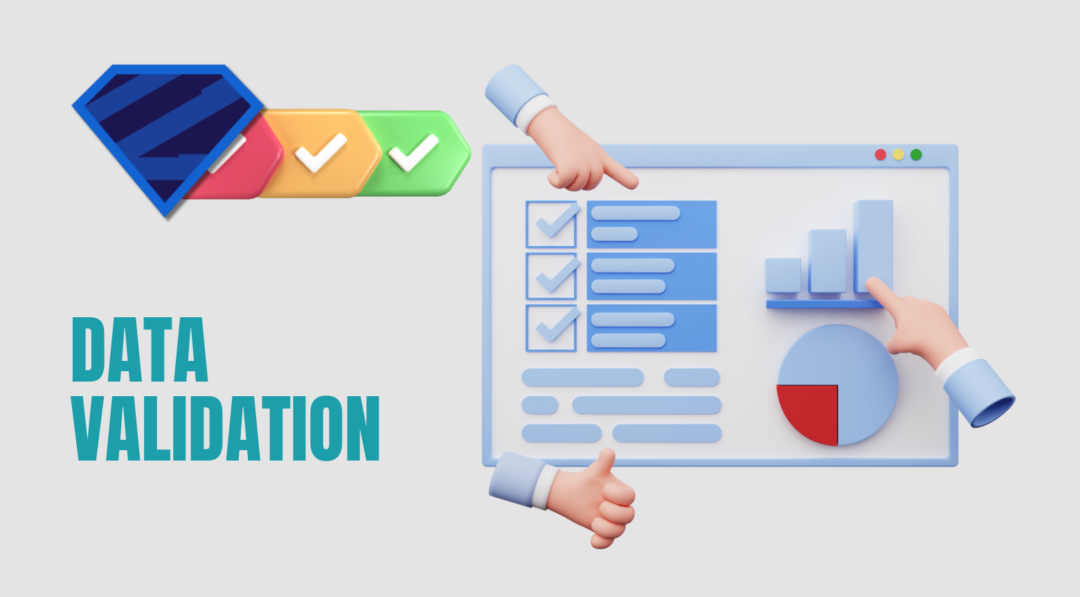
Once you’ve defined your schema, it’s time to validate data. Zod gives you two primary methods for validation:
.parse()
This method parses the data and throws an error if the data doesn’t match the schema. It’s great for explicit error handling and ensures that your application fails fast when invalid data is encountered.
try {
const result = dataSchema.parse(response); // Throws an error if invalid
} catch (error) {
console.error("Invalid data!", error);
}
.safeParse()
This method parses the data but returns an object indicating success or failure without throwing errors. It’s useful when you want to handle errors gracefully or log them without crashing the application.
const result = dataSchema.safeParse(response); // Silent failure
if (!result.success) {
console.error("Invalid data:", result.error);
}
These two methods give you the flexibility to choose how you want to handle validation errors, depending on your specific use case.
Using Zod in Real-Life Scenarios
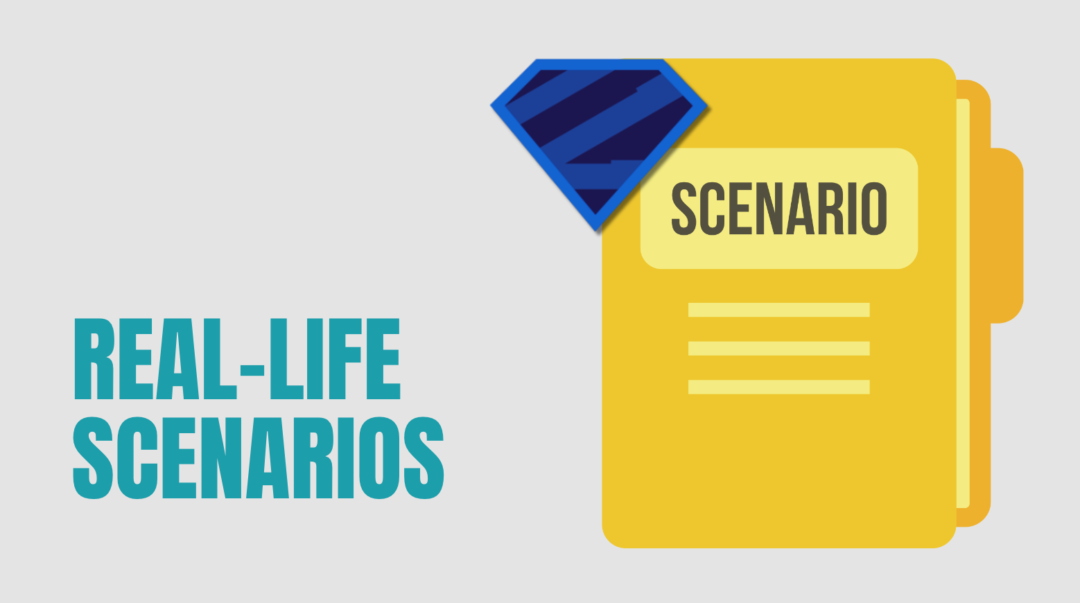
Now that we know how to define and validate schemas, let’s explore some real-world scenarios where Zod shines.
Validating API Responses
const response = await fetchDataFromApi();
const validation = dataSchema.safeParse(response);
if (!validation.success) {
console.error("Invalid API Response:", validation.error);
}
User Form Inputs
User input is another common source of data that needs validation. You can define a Zod schema that validates not just the types, but also the business rules, like string length, number ranges, etc.
const formSchema = z.object({
username: z.string().min(3, "Username must be at least 3 characters long"),
age: z.number().min(18, "You must be at least 18 years old"),
});
try {
const formData = formSchema.parse(userInput); // Will throw if invalid
} catch (error) {
console.error("Invalid form data:", error);
}
Zod allows you to validate user input on the fly and provides detailed error messages that can be used to give users clear feedback.
Custom Validation with .refine()
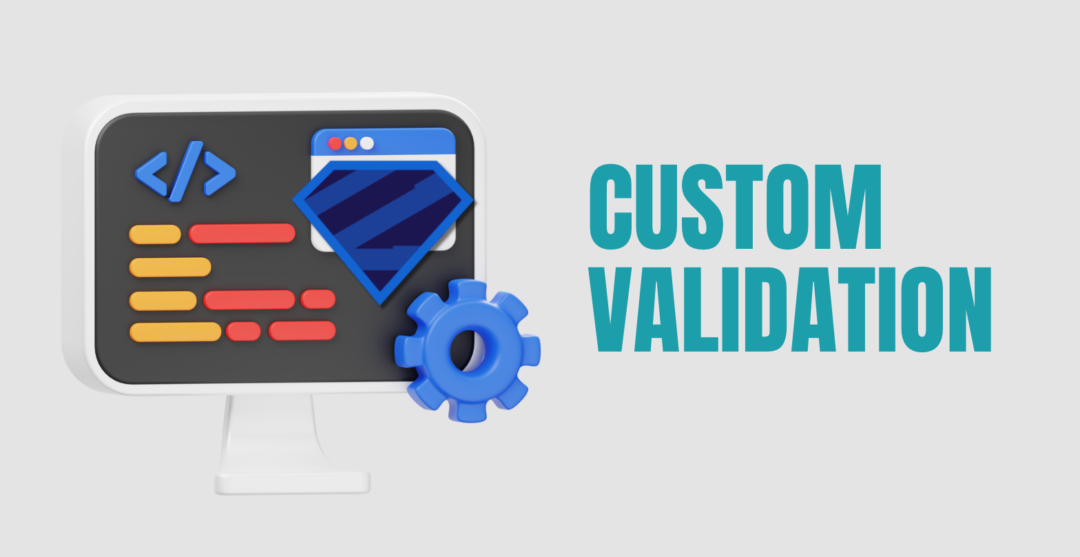
If you need more custom validation logic, you can use .refine()
to add custom checks.
const schema = z.string().refine((val) => val.startsWith('A'), {
message: "String must start with 'A'",
});
try {
schema.parse("Apple"); // Passes
schema.parse("Banana"); // Throws an error
} catch (e) {
if (e instanceof z.ZodError) {
console.log(e.errors);
}
}
Advanced Features in Zod
Zod isn’t just for simple data validation. It comes with several advanced features that can handle complex data validation scenarios:
refine()
: Used for custom validation logic.
const passwordSchema = z.string().refine((val) => /[A-Z]/.test(val), {
message: "Password must contain at least one uppercase letter",
});
multipleOf()
: Ensures a number is a multiple of a given value.
const numberSchema = z.number().multipleOf(5); // Validates that the number is a multiple of 5
- Enums and Literals: You can validate specific values using enums or literals.
const statusSchema = z.nativeEnum(Status); // Validates against a TypeScript enum
Handling Errors
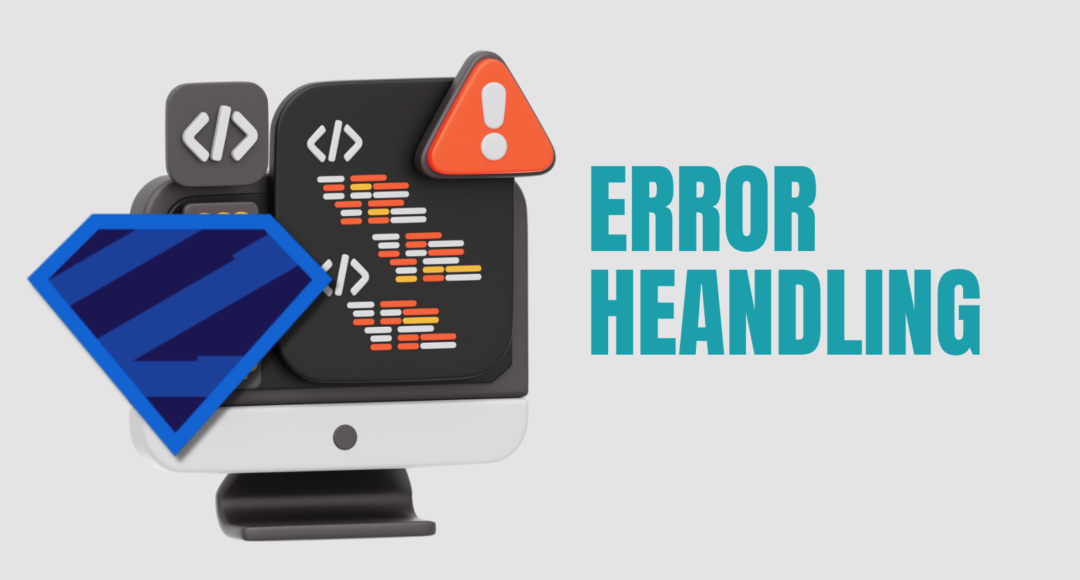
When validation fails, Zod throws an instance of ZodError
. You can handle errors like this:
try {
UserSchema.parse({ name: 'A', age: 15, email: 'invalid-email' });
} catch (error) {
if (error instanceof z.ZodError) {
error.errors.forEach(e => console.log(e.message));
}
}
Final Thoughts and Next Steps
Zod is an excellent choice for frontend developers who want to ensure the runtime validity of external data. By integrating Zod into your application, you can handle complex validation scenarios with ease. Whether you’re validating API responses or user input, Zod provides a straightforward way to define and enforce data schemas.
For further learning, check out these resources:
- Zod Documentation: The official documentation is a fantastic place to start.
- Web Dev Simplified Tutorial: An easy-to-follow guide that breaks down how Zod works in practice.
Embrace Zod to bring an extra layer of safety and validation to your applications. Your users and codebase will thank you!